Step 5
Note the following variables are used to hold the state of the game:
const numRows = 3;
const numCols = 3;
let numEmptyCells = numRows * numCols;
const board = new Array(numEmptyCells);
const markers = ["x", "o"];
let player = 0;
let gameIsOver = false;
The variables above are declared in the script.js
(outside of any functions) and therefore have a global scope. It is generally not a good practice to declare globally scoped variables; we leave it at that for now and return to the topic in later modules.
The remainder of script.js
contains several function definitions. These functions are defined in standard form using a function
keyword, followed by the function name, parameters (if any), and the function body.
Note a JavaScript function can return a value (using the return statement). Unlike functions in Java and C++, functions in JavaScript have no parameter/return types.
Let's look at the function definition of toLinearIndex
as an example:
// return the linear index corresponding to the row and column subscripts
function toLinearIndex(row, col) {
return row * numRows + col;
}
Notice numRows
is defined globally and the function has access to it.
The game board is a 3 x 3 grid:
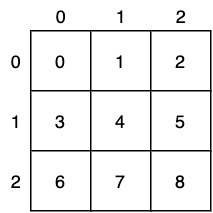
However, we store this board in a 1D array (board
):

The toLinearIndex
function, as the name suggests, returns the linear index into board
corresponding to the row and column subscripts of the game board.